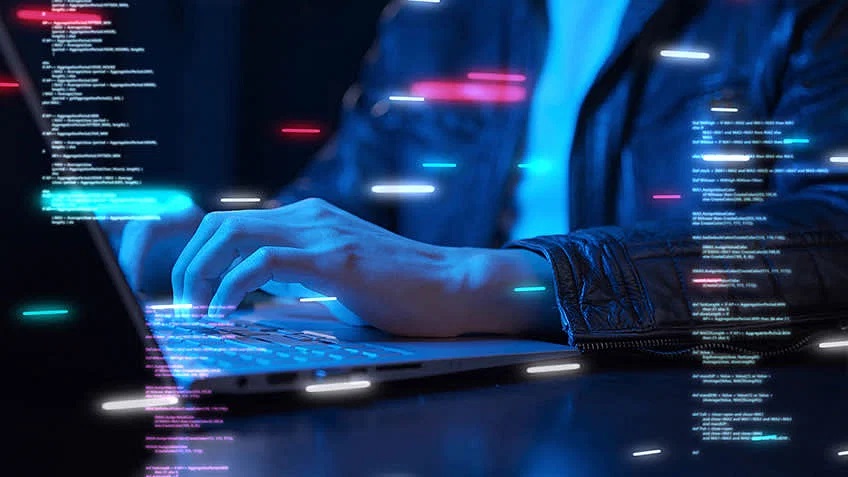
Guide to Preventing Vulnerabilities You Encounter With C Coding
Security in C: Best Practices for Avoiding Common Vulnerabilities
If you’re new to coding, you may only have heard about vulnerabilities of various programming languages in passing. What does vulnerability mean? Technically, that’s any set of conditions that allow a third party to violate an existing security policy, whether implicit or explicit.
Safety is very important in anything we do. Every industry understands this need, even iGaming operators, meaning you never have to worry about your security when playing the 25 euro no deposit bonus at GGbet Casino. Being protected while gambling but vulnerable while coding sounds really strange. So, it is time to finally understand the peculiarities of C. Let’s take a stroll down vulnerability lane.
Best Practices to Avoid Vulnerabilities
If you want to code securely in C, which we strongly advise, you must implement the best practices. This involves safe handling and using better functions. Check out the best C practices.
Use of Safe Standard Library Functions
There are many functions you can use in C programming, but some are considered safer than others. On the long list of those that shouldn’t be used are the strcpy() and strncpy() functions. It is easy to misuse them, giving users with malicious intent access to the functionality of a running program and the ability to change it by way of a buffer overflow attack. Here’s how they both work:
- Strcpy () function copies the source string (src) to the destination string (dest), terminating the NULL character if the buffer size of the dest string is more and keeping the NULL character if it is less. This could be a dangerous function because it does not specify the size of the dest array, posing the risk of copying a large character array into a small one. This would result in the strcpy () to have an undefined behaviour;
- Strncpy () function copies only a certain number of bytes of the source string. If no NULL character is copied, the string pasted in dest will not be NULL terminated. This could be a problem as a non-terminated C string could destroy the entire code eventually.
The primary problem with these functions is that they do not guarantee that the dest string will be NULL terminated. You can use others like strncpy () and snprintf () to prevent buffer overflow.
Memory Management
When you get on your system, you’ll realize that your computer memory is finite and has to be managed. It’s why your system freezes or hangs sometimes when another application or program has used up the resources, and a new request you are running has no free memory to use. So, how does that translate in C?
Allocating memory involves assigning virtual or physical memory space to a program. There are two types of memory allocation: static and dynamic. However, for best practices, you want to stick to dynamic, and here’s why. Unlike static, which allocates a fixed memory during compilation, dynamic allocates at run time, using Heap to manage the memory and giving you more flexibility. You’ll need to use several functions properly to get the best results and prevent memory leaks:
- malloc (): reserves a single block of memory;
- calloc (): reserves multiple blocks of storage;
- free (): releases used-up storage when the program ends;
- realloc (): alters the size of a block.
Avoiding Dangerous Functions
When learning to code with C, you’ll encounter several functions with various uses. The gets () input function is one of such that reads string characters. It is primarily a standard input function, but it has its flaws, which is why we advise you not to use it or at least proceed with caution.
The gets () function lacks bounds checking and the ability to determine the length of the incoming line accurately. Using this function could allow coders with malicious intent to effect changes in the functionality of a running program. It’ll involve a buffer overflow attack.
Secure Coding Standards
There is a reason why standards are set in programming. Before you decide to ignore them, consider the idea behind it. When you adhere to these secure coding standards, you protect yourself and your code from malicious attacks. You’ll also be able to ensure your code quality, reducing your security vulnerabilities. Coding standards like CERT C provide rules and recommendations that would help you reduce risks, eliminating vulnerable codes from the embedded software.
Locking Down C
It’s no use writing lines of code and leaving them to chance. You already know the limitations of the C programming language, so you must go the extra mile to secure your code and prevent attackers from getting their fingerprints all over it. You can use C effectively once you’ve got these best practices down, so implement them and research further to tighten your hold on your programming skills. Have you ever had such issues? We’d love to hear about them.